亲爱的游戏爱好者们,你是否曾梦想过亲手打造一款属于自己的游戏?别再羡慕那些游戏大作的开发者了,今天,就让我带你一起走进游戏代码的世界,揭开它的神秘面纱!
一、初识游戏代码:从猜数字游戏开始
还记得小时候玩过的猜数字游戏吗?简单又有趣,其实,这就是一个典型的游戏代码案例。下面,就让我们用C语言来写一个猜数字游戏,感受一下游戏代码的魅力吧!
```c
include
include
include
int main() {
int num, guess, count = 0;
srand(time(0)); // 用系统时间初始化随机种子
num = rand() % 100 + 1; // 生成1-100之间的随机数字
printf(\猜数字游戏开始,数字已生成。\
do {
printf(\请输入你的猜测(1-100):\);
scanf(\%d\, &guess);
count++; // 记录猜测次数
if (guess > num)
printf(\猜大了,请继续猜测。\
else if (guess < num)
printf(\猜小了,请继续猜测。\
else
printf(\恭喜你,猜对了!总共猜了%d次。\
\, count);
} while (guess != num);
return 0;
这段代码是不是很简单?其实,游戏代码的魅力就在于它的简洁和高效。通过简单的逻辑判断,就能实现一个有趣的游戏。
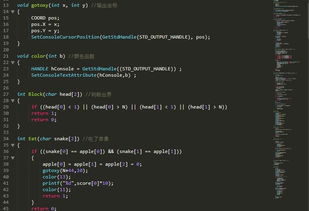
二、游戏代码的进阶:从猜数字到贪吃蛇
当你掌握了猜数字游戏的代码后,是不是已经跃跃欲试,想要挑战更复杂的游戏呢?接下来,我们就来尝试编写一个经典的贪吃蛇游戏。
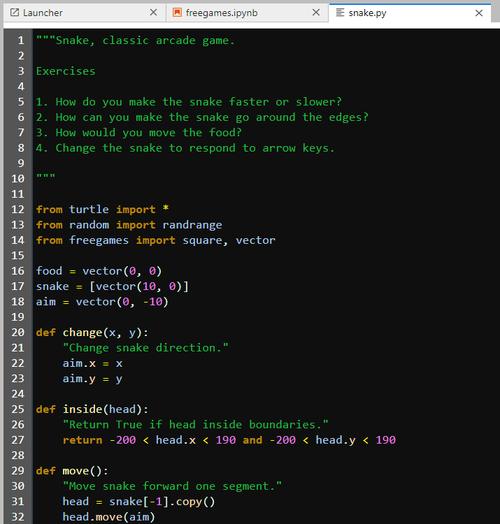
贪吃蛇游戏需要用到很多游戏编程的知识,比如图形界面、事件处理、碰撞检测等。下面,就让我们用Python来写一个简单的贪吃蛇游戏,感受一下游戏代码的进阶之旅。
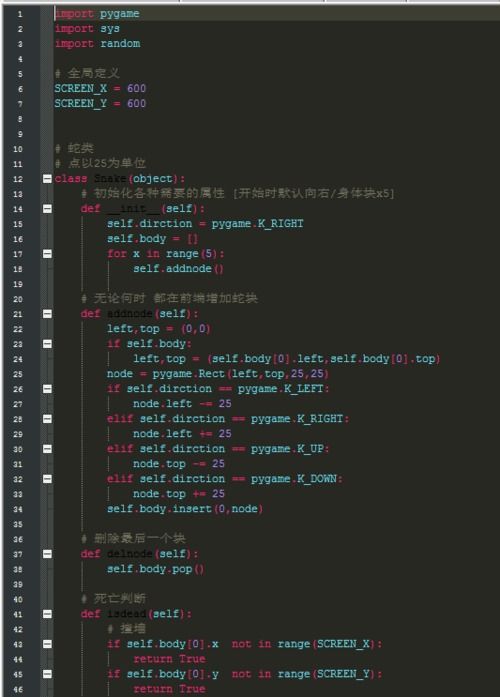
```python
import turtle
import time
import random
设置屏幕
wn = turtle.Screen()
wn.title(\贪吃蛇游戏\)
wn.bgcolor(\black\)
wn.setup(width=600, height=600)
wn.tracer(0)
创建蛇头
head = turtle.Turtle()
head.speed(0)
head.shape(\square\)
head.color(\white\)
head.penup()
head.goto(0, 0)
head.direction = \stop\
创建蛇食
food = turtle.Turtle()
food.speed(0)
food.shape(\circle\)
food.color(\red\)
food.penup()
food.goto(0, 100)
segments = []
创建分数板
score_board = turtle.Turtle()
score_board.speed(0)
score_board.shape(\square\)
score_board.color(\white\)
score_board.penup()
score_board.hideturtle()
score_board.goto(0, 260)
score_board.write(\得分:0\, align=\center\, font=(\Courier\, 24, \normal\))
控制蛇头移动
def go_up():
if head.direction != \down\:
head.direction = \up\
def go_down():
if head.direction != \up\:
head.direction = \down\
def go_left():
if head.direction != \right\:
head.direction = \left\
def go_right():
if head.direction != \left\:
head.direction = \right\
def move():
if head.direction == \up\:
y = head.ycor()
head.sety(y + 20)
if head.direction == \down\:
y = head.ycor()
head.sety(y - 20)
if head.direction == \left\:
x = head.xcor()
head.setx(x - 20)
if head.direction == \right\:
x = head.xcor()
head.setx(x + 20)
键盘绑定
wn.listen()
wn.onkeypress(go_up, \w\)
wn.onkeypress(go_down, \s\)
wn.onkeypress(go_left, \a\)
wn.onkeypress(go_right, \d\)
主游戏循环
while True:
wn.update()
检查是否撞墙
if head.xcor() > 290 or head.xcor() < -290 or head.ycor() > 290 or head.ycor() < -290:
time.sleep(1)
head.goto(0, 0)
head.direction = \stop\
隐藏蛇身
for segment in segments:
segment.goto(1000, 1000)
segments.clear()
重置分数
score = 0
score_board.clear()
score_board.write(\得分:{} \.format(score), align=\center\, font=(\Courier\, 24, \normal\))
检查是否吃到食物
if head